How to Manage Environment Variables in Node.js with dotenv
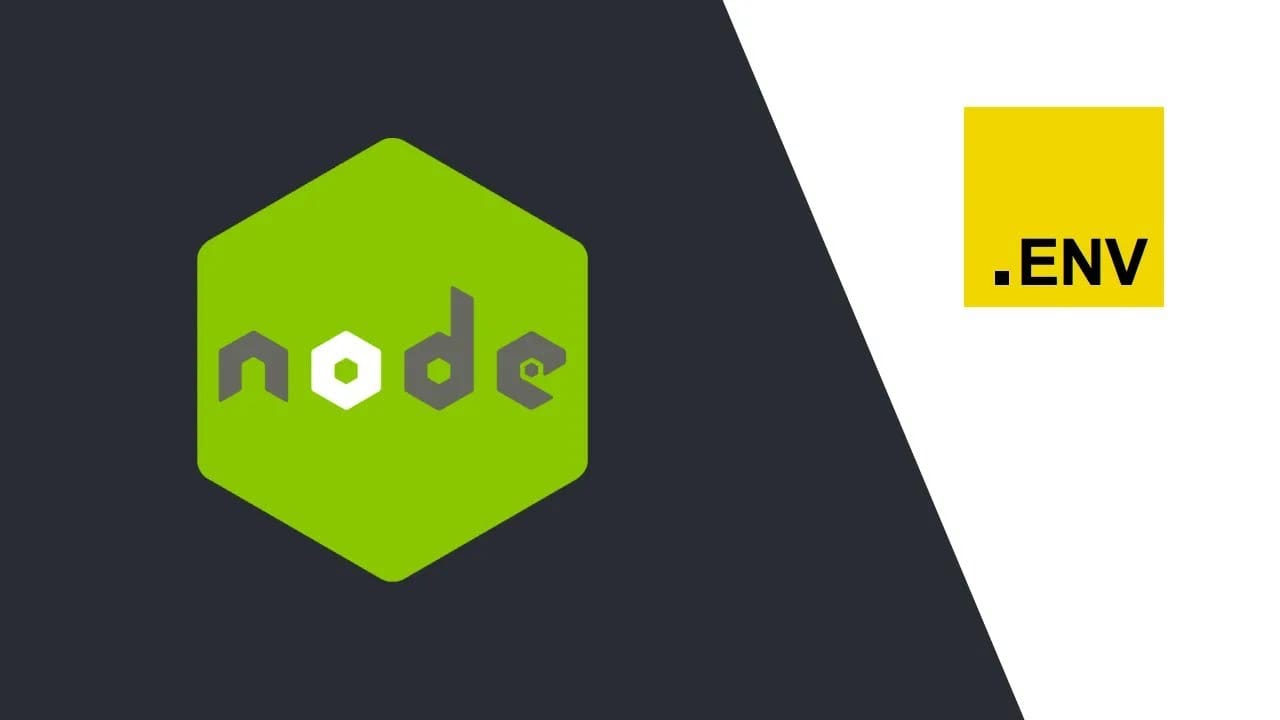
In modern software development, managing configurations, sensitive data, and settings for various environments (like development, staging, and production) is essential for building secure and scalable applications.
One of the best practices to handle this in Node.js applications is by using environment variables.
dotenv is a popular npm package that helps developers manage environment variables in a straightforward and efficient way. In this article, we will explore what dotenv
is, why it is important, and how to use it in your Node.js applications.
What is dotenv
dotenv
is a zero-dependency Node.js package that loads environment variables from a .env
file into process.env
.
It makes it easy to manage sensitive information like API keys, database credentials, and other configuration values outside your codebase.
This way, you avoid hardcoding sensitive data and can easily switch configurations for different environments.
Why Use dotenv
- Separation of Concerns: Storing configuration values (like API keys or database passwords) directly in your code can lead to security issues. By using environment variables, you can separate configuration from your application logic.
- Environment-Specific Configuration: You can create different
.env
files for different environments (e.g.,.env.development
,.env.production
) and load the appropriate configuration depending on the environment. - Security: Hardcoding sensitive data in the codebase can make it vulnerable. With
dotenv
, sensitive data is stored outside of your code, often in environment files, and can be safely excluded from version control (e.g., Git). - Easy Setup and Use:
dotenv
is simple to set up and provides a clean and easy-to-read way to manage configuration values.
How to Install and Use dotenv
Here’s how to integrate dotenv
into your Node.js project:
- Install
dotenv
- Start by installing the
dotenv
package in your Node.js project.- Open your terminal and navigate to your project directory.
- Run the following command to install
dotenv
via npm:
- Start by installing the
npm install dotenv
- Create a
.env
File
- In the root of your project, create a file called
.env
. - This file will contain your environment variables in the
KEY=VALUE
format.
- Example of a
.env
file:
DB_HOST=localhost
DB_PORT=27017
DB_USER=myuser
DB_PASSWORD=mypassword
API_KEY=myapikey
PORT=3000
- The keys are the names of your environment variables, and the values are their respective settings.
- Important: Never commit
.env
files to version control (e.g., Git) because they often contain sensitive data. Use a.gitignore
file to exclude.env
from being tracked.
Load Environment Variables in Your Application
- Now that you’ve installed
dotenv
and created a.env
file, you need to load these variables into your application. - In your main JavaScript file (e.g.,
app.js
,server.js
), add the following line at the very top:
require('dotenv').config();
- This line of code tells
dotenv
to load the environment variables defined in the.env
file intoprocess.env
.
Example:
// Load environment variables from .env file
require('dotenv').config();
// Use environment variables in your application
const port = process.env.PORT || 3000;
const dbHost = process.env.DB_HOST;
const dbPort = process.env.DB_PORT;
console.log(`Server is running on port: ${port}`);
console.log(`Connecting to database at ${dbHost}:${dbPort}`);
In the example above:
process.env.PORT
retrieves thePORT
variable from the.env
file (if it’s defined).- If the
PORT
variable isn’t defined, it defaults to3000
. - Other variables such as
DB_HOST
andDB_PORT
are used similarly.
Accessing Environment Variables
- Once the
.env
file is loaded, you can access the variables throughout your application usingprocess.env
.
For example:
process.env.DB_HOST
accesses the value of theDB_HOST
variable from.env
.process.env.API_KEY
retrieves your API key.
Important Notes:
- All environment variables loaded using
dotenv
are strings, even if they represent numbers or booleans. - For types like numbers or Booleans, you may need to cast them:
const port = parseInt(process.env.PORT, 10); const isDebug = process.env.DEBUG === 'true'; // Convert 'true'/'false' to boolean
Exclude .env
from Version Control
Since .env
files often contain sensitive information, you should make sure it is excluded from version control (like Git).
- Create a
.gitignore
file in the root of your project if it doesn’t already exist. - Add the following line to
.gitignore
:.env
This will ensure that .env
files are not tracked by Git and do not end up in your repository.
Best Practices with dotenv
- Multiple Environment Files
- Use different
.env
files for different environments (development, testing, production). You can specify which file to load usingdotenv
‘spath
option.
- Use different
require('dotenv').config({ path: '.env.production' });
- Avoid Hardcoding Values
- Never hardcode sensitive values like passwords or API keys directly in your code. Always use environment variables.
- Use Default Values
- For non-sensitive configuration, provide default values in the code when accessing
process.env
, so the application doesn’t crash if a variable is missing:
- For non-sensitive configuration, provide default values in the code when accessing
const dbHost = process.env.DB_HOST || 'localhost';
- Keep
.env
Files Secure- Store
.env
files in a secure location, especially in production environments. Ensure that only authorized individuals have access to them.
- Store
- Validate Environment Variables
- Consider using packages like
joi
orenvalid
to validate the presence and correctness of critical environment variables.
- Consider using packages like
dotenv
is a powerful and easy-to-use tool that simplifies managing environment variables in Node.js applications.
It helps to keep sensitive data out of the source code, supports multiple environments, and follows best practices for secure and scalable app configuration.
By following the steps outlined in this guide, you can easily integrate dotenv
into your Node.js projects and start using environment variables to manage your app’s configuration in a secure and maintainable way.
Happy Coding !