Real Time User Analytics API: Track Device, Geolocation, and Proxy Data with Node.js and MongoDB
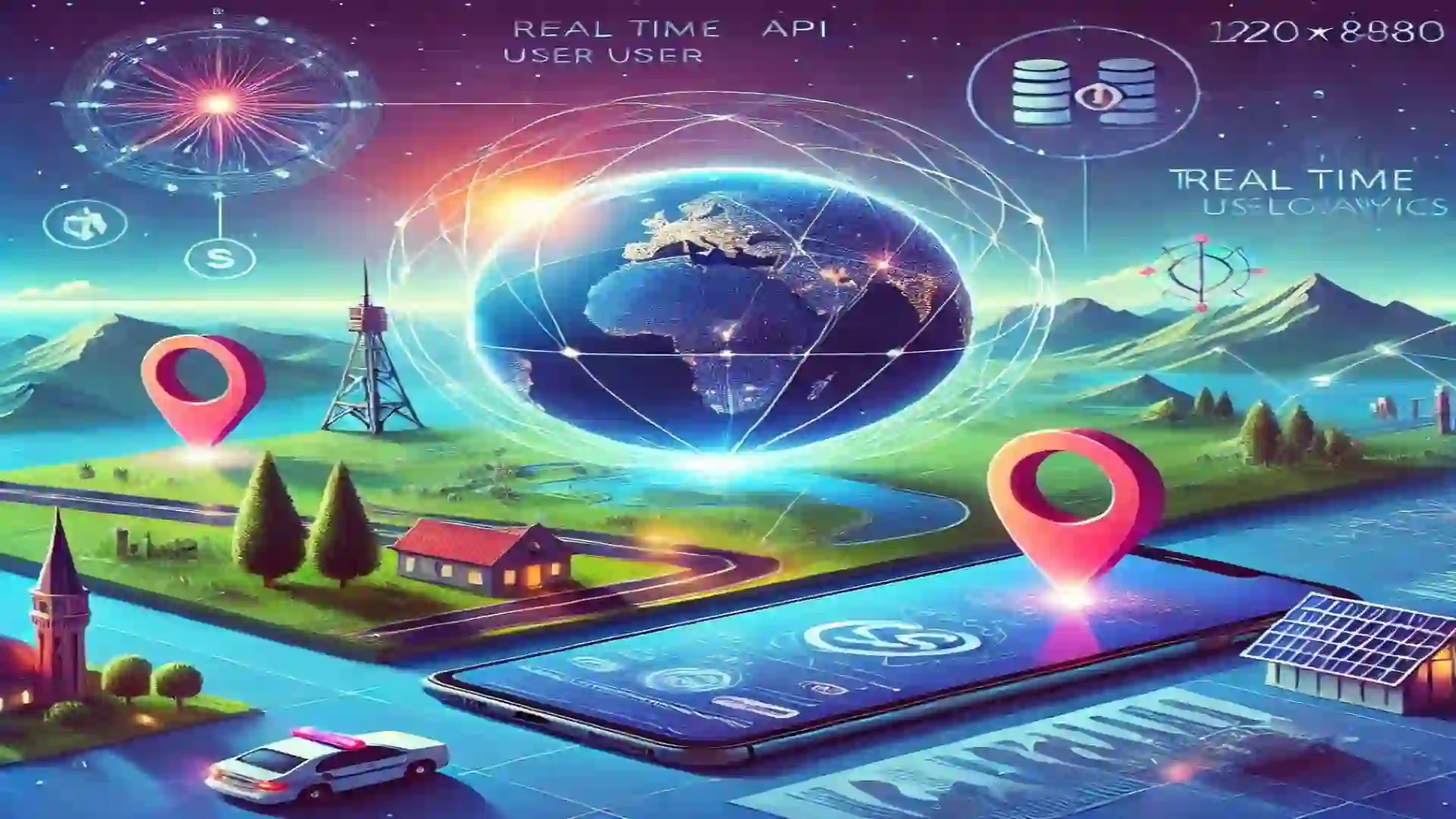
This project delivers a robust API for real-time user analytics, built with Node.js and MongoDB.
The API actively collects detailed user data, including device information (browser and OS), IP address, geolocation, and proxy detection status.
It securely stores this data in a MongoDB database for easy retrieval and analysis.
With a focus on accuracy and performance, the API enables businesses to identify real visitors, detect proxy users, and gain insights into user behavior.
The scalable design ensures seamless handling of large datasets, making it ideal for analytics, security, or marketing applications.
Perfect for developers and organizations seeking to track and analyze user activity effectively in real time.
What Is a Real-Time User Analytics API?
A Real-Time User Analytics API tracks and collects user data as they interact with your system or website. The data includes:
- Device Information: Browser type, operating system (OS).
- Geolocation: Country, city, and region based on the IP address.
- IP Address and Network Data: Identifies unique IPs and detects if users access the service via proxies or VPNs.
- Timestamp: Logs the exact time of the user’s visit.
This API is essential for:
- Monitoring genuine users and detecting suspicious traffic.
- Gaining insights into the geographic and device distribution of users.
- Improving application security with proxy detection.
Technology Stack
To implement this project, we use:
- Node.js: A fast and scalable runtime for building the backend.
- Express.js: Simplifies the creation of HTTP APIs.
- MongoDB: A NoSQL database for storing user analytics data.
- GeoIP-lite: Provides geolocation information based on IP addresses.
- Express-useragent: Extracts user agent details such as browser and OS.
- IPQualityScore (optional): Detects proxy or VPN usage.
Core Features of the API
1. Data Collection
The API collects essential details from every request:
- The user’s IP address is identified via request headers.
- Browser and OS details are extracted from the user agent.
- Geolocation data (city, region, country) is retrieved using
geoip-lite
.
2. Proxy Detection
Using IP-based services like IP Quality Score, the API determines if a user is accessing via a proxy, VPN, or TOR.
3. Data Storage
The collected data is stored in MongoDB, with a schema including:
- IP address
- Browser and OS
- Geolocation
- Proxy status
- Timestamp
4. Dashboard Integration
The API supports integration with dashboards, allowing data visualization for traffic analysis and trends.
Step-by-Step Implementation
1. Initialize the Project
Run the following commands to set up your project:
mkdir user-data-api
cd user-data-api
npm init -y
npm install express mongoose geoip-lite express-useragent cors dotenv
2. Configure ES6 Modules
Add the following to your package.json
:
"type": "module"
3. Project Structure
user-data-api/
├── src/
│ ├── config/
│ │ └── db.js # MongoDB connection setup
│ ├── models/
│ │ └── User.js # Mongoose schema
│ ├── routes/
│ │ └── userRoutes.js # API routes
│ ├── controllers/
│ │ └── userController.js # Logic to handle requests
│ └── index.js # Main entry point
├── .env # Environment variables
└── package.json
4. Code Implementation
4.1. MongoDB Configuration (src/config/db.js
)
import mongoose from "mongoose";
const connectDB = async () => {
try {
const conn = await mongoose.connect(process.env.MONGO_URI);
console.log(`MongoDB Connected: ${conn.connection.host}`);
} catch (error) {
console.error(`Error: ${error.message}`);
process.exit(1);
}
};
export default connectDB;
4.2. User Model (src/models/User.js
)
import mongoose from "mongoose";
const userSchema = new mongoose.Schema({
ip: { type: String, required: true },
browser: { type: String },
os: { type: String },
location: { type: Object },
timestamp: { type: Date, default: Date.now },
});
const User = mongoose.model("User", userSchema);
export default User;
4.3. User Controller (src/controllers/userController.js
)
import geoip from "geoip-lite";
import User from "../models/User.js";
export const logUserData = async (req, res) => {
try {
const ip = req.headers["x-forwarded-for"] || req.connection.remoteAddress;
const { browser, os } = req.useragent;
const location = geoip.lookup(ip);
// User data to save
const userData = { ip, browser, os, location };
const user = new User(userData);
await user.save();
res.status(200).json({ message: "User data logged successfully", userData });
} catch (error) {
res.status(500).json({ message: "Error logging user data", error });
}
};
export const getAllUsers = async (req, res) => {
try {
const users = await User.find().sort({ timestamp: -1 });
res.status(200).json(users);
} catch (error) {
res.status(500).json({ message: "Error fetching user data", error });
}
};
4.4. Routes (src/routes/userRoutes.js
)
import express from "express";
import { logUserData, getAllUsers } from "../controllers/userController.js";
import useragent from "express-useragent";
const router = express.Router();
// Middleware to extract user agent data
router.use(useragent.express());
// Route to log user data
router.get("/", logUserData);
// Route to fetch all users
router.get("/users", getAllUsers);
export default router;
4.5. Main Entry Point (src/index.js
)
import express from "express";
import cors from "cors";
import dotenv from "dotenv";
import connectDB from "./config/db.js";
import userRoutes from "./routes/userRoutes.js";
dotenv.config();
// Connect to MongoDB
connectDB();
const app = express();
// Middleware
app.use(cors());
app.use(express.json());
// Routes
app.use("/api", userRoutes);
// Start Server
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => console.log(`Server running on port ${PORT}`));
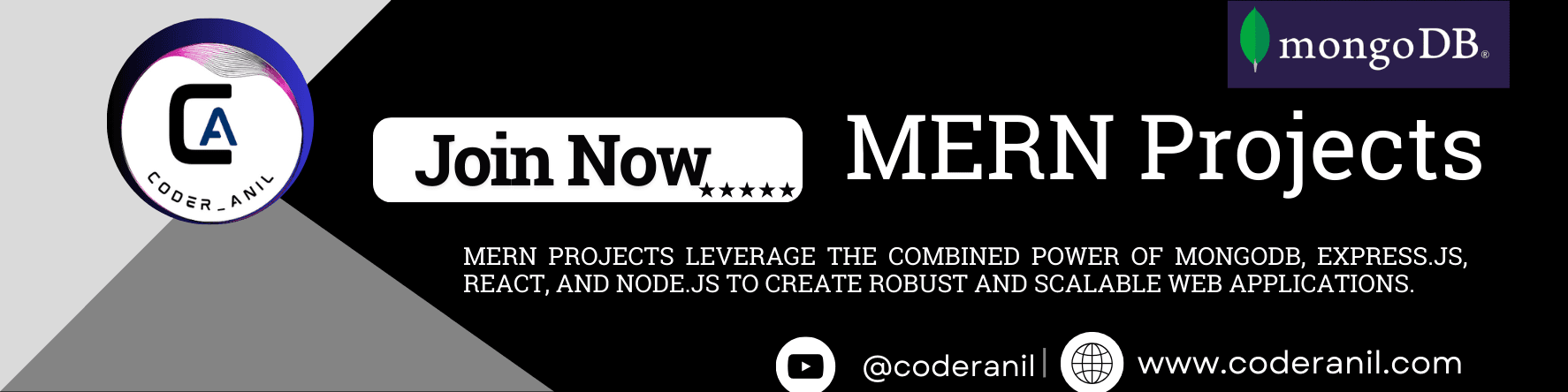
5. Environment Variables
Create a .env
file in the root directory:
MONGO_URI=your_mongodb_connection_string
6. Run the Application
- Start your MongoDB instance (e.g., via Atlas or locally).
- Run the server:
node src/index.js
API Endpoints
- Log User Data:
GET /api/
- Collects user data and logs it in the database.
- Get All Users:
GET /api/users
- Retrieves all logged user data.
Building a Real-Time User Analytics API with Node.js and MongoDB empowers you to gather actionable data while maintaining a secure and scalable system.
This solution is perfect for organizations looking to monitor traffic, detect proxies, and gain deeper insights into user behavior.
Start implementing this project today and unlock the full potential of real-time analytics for your applications!
Happy coding !