How to Manage Environment Variables using ES6 in Node.js with dotenv
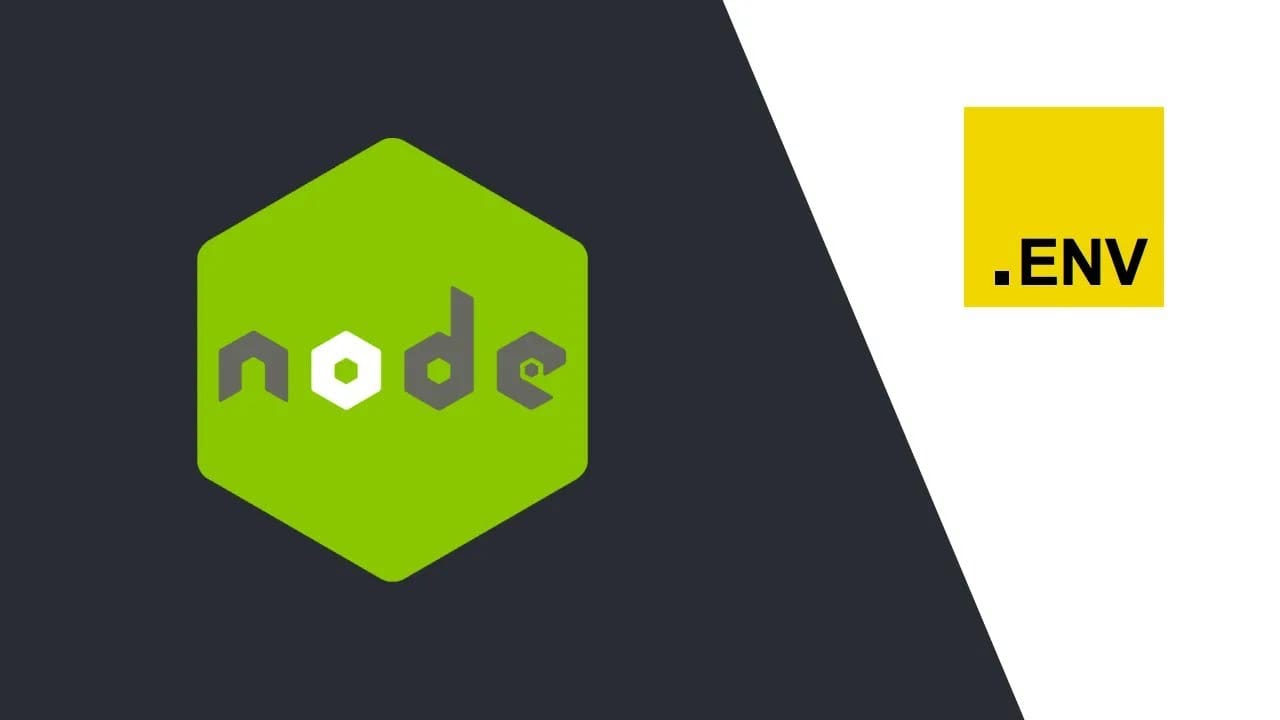
In modern web development, handling sensitive data like API keys, database credentials, or secret tokens securely is crucial. A popular method for managing such environment variables is by using .env
files. In this article, we will explore how to use the dotenv package in a Node.js environment with ES6 Modules (ESM).
What is dotenv?
The dotenv
package allows you to load environment variables from a .env
file into process.env
in Node.js. This approach helps keep sensitive information out of your codebase and version control, making it safer and easier to manage configurations across different environments (development, staging, production, etc.).
Setting Up a Node.js Project with ES6 Modules
Before we start using the dotenv
package, we need to set up a Node.js project that uses ES6 Modules (import/export).
- Initialize a Node.js Project
- Open your terminal and run the following command to create a new project:
mkdir my-dotenv-project cd my-dotenv-project npm init -y
- Enable ES6 Modules
- In your
package.json
file, add the following to enable ES6 module support:
{ "type": "module" }
- Install dotenv Package
- Now, install the
dotenv
package via npm:
npm install dotenv
Creating and Configuring the .env
File
The .env
file stores your environment variables in a key-value format. Here’s how to create and configure it:
- Create a
.env
File - In the root of your project, create a file named
.env
- Add Environment Variables
- Add key-value pairs representing your environment variables. For example:
API_KEY=your-api-key-here DB_PASSWORD=your-database-password-here SECRET_TOKEN=your-secret-token-here
- Important: Ensure that
.env
is added to your.gitignore
file to prevent it from being committed to version control.
Using dotenv with ES6 Modules
To load the environment variables defined in the .env
file into your Node.js project using ES6 Modules, follow these steps:
- Create Your JavaScript File
- Create an
index.js
file ( or any JavaScript file where you want to use the environment variables).
- Import dotenv and Load Variables
- In your
index.js
, use the ES6import
syntax to import thedotenv
package and load the environment variables In this code:
import dotenv from 'dotenv';
// Load environment variables from .env file
dotenv.config();
// Access environment variables using
const apiKey = process.env.API_KEY; process.env
const dbPassword = process.env.DB_PASSWORD;
const secretToken = process.env.SECRET_TOKEN;
// Log the variables (for testing purposes)
console.log('API Key:', apiKey);
console.log('Database Password:', dbPassword);
console.log('Secret Token:', secretToken);
dotenv.config()
loads the environment variables from the.env
file intoprocess.env
.
- You can then access each environment variable using
process.env.VARIABLE_NAME
.
Run Your Application
To test if it works, run the following command in your terminal: node index.js
This should output the values from your .env
file (if you’ve set them correctly).
Best Practices for Using dotenv
- Keep .env File Secret
- Always ensure that your
.env
file is not committed to version control (e.g., Git). To do this, add.env
to your.gitignore
file:.env
- Use Default Values with dotenv
- It’s a good practice to set default values for critical environment variables to ensure your application doesn’t break if a variable is missing. You can achieve this like so:
const apiKey = process.env.API_KEY || 'default-api-key'; const dbPassword = process.env.DB_PASSWORD || 'default-db-password';
- Use Descriptive Names
- Name your environment variables descriptively to make them easy to understand for other developers who may work with your codebase.
Use Different .env Files for Different Environments
- You can create multiple
.env
files for different environments (e.g.,.env.development
,.env.production
). Use a tool likecross-env
or configure your build system to load the correct.env
file depending on the environment.
Example: Building a Simple App Using dotenv
- Let’s create a simple app that connects to a database (we will just simulate this with a console log) using the environment variables.
- Update
.env
- Add the following to your
.env
file:
DB_HOST=localhost DB_USER=root DB_PASSWORD=secretpassword
- Create
index.js
import dotenv from 'dotenv'; dotenv.config(); const dbHost = process.env.DB_HOST; const dbUser = process.env.DB_USER; const dbPassword = process.env.DB_PASSWORD; // Simulate a database connection console.log(`Connecting to database at ${dbHost}...`); console.log(`Using user: ${dbUser}`); console.log(`Password: ${dbPassword}`);
- Run the App
- Run the application with:
node index.js
The output should look like:Connecting to database at localhost... Using user: root Password: secretpassword
Using dotenv
with ES6 modules in Node.js is a great way to manage sensitive data and environment-specific configurations. By keeping your credentials and configuration separate from your code, you ensure better security, flexibility, and maintainability in your applications. With the steps outlined above, you should be able to easily set up dotenv
in your Node.js project and start using environment variables for various purposes.
Happy coding!