How to Build a Password Generator Using React, Vite, and Tailwind CSS
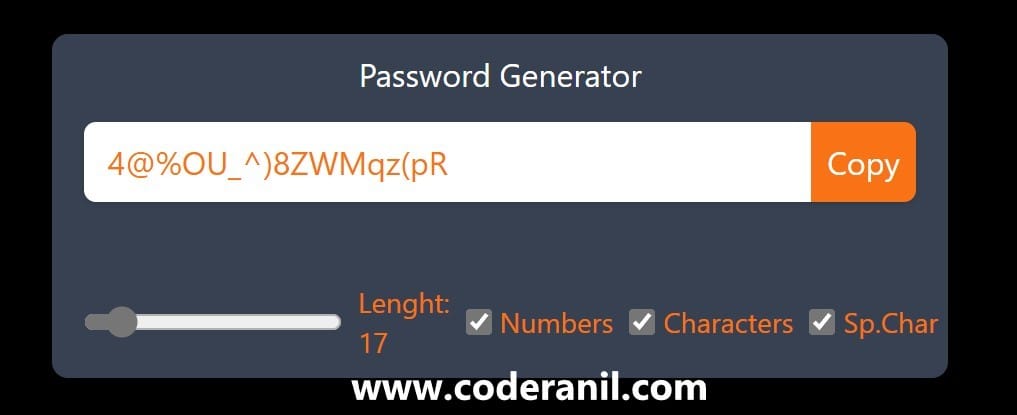
Creating a password generator in React is a great way to practice your skills in JavaScript and React. In this article, we will walk through the steps to build a simple password generator app using Vite and Tailwind CSS for better performance and modern styling. By the end, you will have a functional app that can create secure passwords.
Prerequisites
Before starting, make sure you have the following:
- Basic knowledge of JavaScript and React.
- Node.js installed on your computer.
- A code editor like VS Code.
Set Up Your Project with Vite
- Open your terminal and create a new Vite app:
npm create vite@latest password-generator --template react
- Navigate to the project folder:
cd password-generator
- Install the dependencies:
npm install
- Start the development server:
npm run dev
This will open the default Vite React app in your browser.
Install and Configure Tailwind CSS
- Install Tailwind CSS and its dependencies:
npm install -D tailwindcss postcss autoprefixer
- Initialize the Tailwind CSS configuration:
npx tailwindcss init -p
- Update
tailwind.config.js
with the following content:
/** @type {import(\'tailwindcss\').Config} */
export default {
content: [
\"./index.html\",
\"./src/**/*.{js,ts,jsx,tsx}\",
],
theme: {
extend: {},
},
plugins: [],
}
- Add Tailwind directives to your
src/index.css
file:
@tailwind base;
@tailwind components;
@tailwind utilities;
- Start the development server if it isn’t already running:
npm run dev
Project Structure
- Your project directory should now look like this:
password-generator/
├── node_modules/
├── public/
│ ├── vite.svg
│ └── index.html
├── src/
│ ├── components/
│ │ └── PasswordGenerator.jsx
│ ├── App.jsx
│ ├── index.css
│ ├── main.jsx
├── .gitignore
├── package.json
├── postcss.config.cjs
├── tailwind.config.js
├── vite.config.js
└── README.md
Clean Up the Default Files
- Open the project in your code editor.
- Delete the contents of
App.css
and replaceApp.jsx
with the following starter code:
function App() {
return (
<div className=\"w-full max-w-md mx-auto shadow-md rounded-lg px-4 py-2 my-20 text-orange-500 bg-gray-700\"\">
<h1 className=\"text-white text-center pb-3\">Password Generator</h1>
</div>
);
}
export default App;
Create the Password Generator Component
- Create a new folder for components:
mkdir src/components
- Create a new file named
PasswordGenerator.jsx
insidesrc/components
and update this code:
import { useState, useCallback, useEffect } from \"react\";
function PasswordGenerator() {
const [length, setLength] = useState(10);
const [numAllow, setNumAllow] = useState(true);
const [charAllow, setCharAllw] = useState(true);
const [specialAllow, setSpecialAllow] = useState(true);
const [Password, setPassword] = useState(" ");
const passwordGen = useCallback(() => {
const num = \"0123456789\";
const char = \"ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz\";
const special = \"!@#$%^&*()_+\";
let mixpass = \"\";
let pass = \"\";
if (numAllow) mixpass += num;
if (charAllow) mixpass += char;
if (specialAllow) mixpass += special;
for (let i = 1; i <= length; i++) {
pass += mixpass.charAt(Math.floor(Math.random() * mixpass.length));}
setPassword(pass);
}, [specialAllow, length, numAllow, charAllow, setPassword]);
useEffect(() => {
passwordGen();
}, [specialAllow, length, numAllow, charAllow, passwordGen]);
return (
<div className=\"flex shadow rounded-md overflow-hidden mb-10 \">
<input
type=\"text\"
value={Password}
className=\"outline-none w-full py-1 px-3\"
placeholder=\"your password\"
readOnly />
<button className=\"bg-orange-500 text-white p-2\">Copy</button>
</div>
<div className=\"flex text-sm gap-x-2\">
<input
type=\"range\"
min={8}
max={100}
value={length}
className=\"cursor-pointer \"
onChange={(e) => setLength(e.target.value)}/>
<label>Lenght: {length}</label>
<div className=\"flex items-center gap-x-1\">
<input
type=\"checkbox\"
defaultChecked={numAllow}
id=\"numberInput\"
onChange={() => {
setNumAllow((prev) => !prev);}}/>
<label>Numbers</label>
</div>
<div className=\"flex items-center gap-x-1\">
<input
type=\"checkbox\"
defaultChecked={charAllow}
id=\"charInput\"
onChange={() => {
setCharAllw((prev) => !prev);}}/>
<label>Characters</label>
</div>
<div className=\"flex items-center gap-x-1\">
<input
type=\"checkbox\"
defaultChecked={specialAllow}
id=\"spicalInput\"
onChange={() => {
setSpecialAllow((prev) => !prev);}}/>
<label>Sp.Char</label>
</div>
</div> )
}
export default PasswordGenerator;
Update the App Component
- Import the
PasswordGenerator
component intoApp.jsx
:
function App() {
return (
<div className=\"w-full max-w-md mx-auto shadow-md rounded-lg px-4 py-2 my-20 text-orange-500 bg-gray-700\"\">
<h1 className=\"text-white text-center pb-3\">Password Generator</h1>
<PasswordGenerator />
</div>
);
}
export default App;
Test Your App
- Save all your files.
- Start the development server if it isn’t already running:
npm run dev
- Open the app in your browser (the Vite server should provide the local URL).
- Test the app by entering a password length and clicking the \”Generate Password\” button. You should see a random password appear below the button.
Finally
You have successfully built a password generator using React, Vite, and Tailwind CSS! This project is a great way to practice modern web development techniques. Keep experimenting and adding features to make the app even better.
Happy Coding !