How to Use the Context API in React: Best Practices and Examples
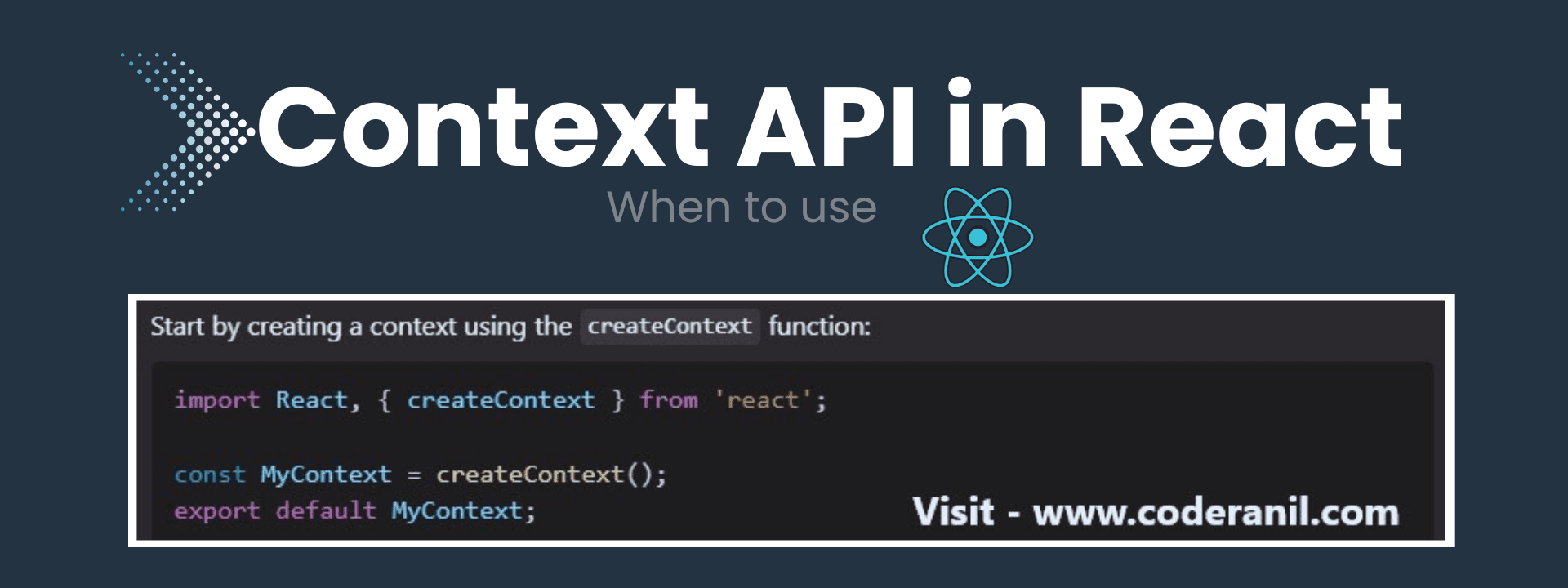
The Context API in React is a powerful feature that simplifies state management and data sharing in React applications. By providing a way to share data across the component tree without passing props manually at every level, the Context API helps streamline the development process. In this article, we’ll explore what the Context API is, when to use it, and how to implement it effectively.
What is the Context API
- The Context API is a feature introduced in React 16.3 that allows developers to share values like state, themes, or other configurations between components without the need for prop drilling.
- Prop drilling, which involves passing props through multiple layers of components, can lead to messy and hard-to-maintain code.
- The Context API addresses this issue by creating a shared context accessible by any component.
When to Use the Context API
While the Context API is powerful, it’s not always the best solution for every scenario. Here are some use cases where it shines:
Theming
- Managing light and dark mode or other design-related themes.
User Authentication
- Sharing user login status and details across components.
Global State Management
- When you need to manage global states like language preferences or cart data in an e-commerce app.
Avoiding Prop Drilling
- When a piece of data needs to be accessed by deeply nested components.
- For more complex state management requirements, libraries like Redux or MobX may be better suited.
How to Implement the Context API
Implementing the Context API in a React application involves three key steps:
Creating a Context
- Start by creating a context using the create Context function:
import React, { createContext } from 'react';
const MyContext = createContext();
export default MyContext;
Providing the Context
- Wrap the components that need access to the context with a Provider component.
- The Provider component makes the context available to all child components.
import React, { useState } from 'react';
import MyContext from './MyContext';
const MyProvider = ({ children }) => {
const [value, setValue] = useState("Hello, Context API!");
return (
<MyContext.Provider value={{ value, setValue }}>
{children}
</MyContext.Provider>
);
};
export default MyProvider;
Consuming the Context
- Use the useContext hook to access the context values in functional components:
import React, { useContext } from 'react';
import MyContext from './MyContext';
const MyComponent = () => {
const { value, setValue } = useContext(MyContext);
return (
<div>
<p>{value}</p>
<button onClick={() => setValue("Context API is awesome!")}>Change Value</button>
</div>
);
};
export default MyComponent;
Complete Example
- Here’s a complete example combining all the steps:
import React from 'react';
import ReactDOM from 'react-dom';
import MyProvider from './MyProvider';
import MyComponent from './MyComponent';
const App = () => (
<MyProvider>
<MyComponent />
</MyProvider>
);
ReactDOM.render(<App />, document.getElementById('root'));
Benefits of Using the Context API
- Simplifies State Sharing: Eliminates the need for prop drilling.
- Built-in Solution: No need to install external libraries.
- Improves Readability: Creates cleaner and more maintainable code.
Conclusion
The Context API in React is an invaluable tool for managing shared state in your applications. By understanding how and when to use it, you can create efficient, scalable, and maintainable React projects. Start implementing the Context API today to experience its full potential.
Happy Coding!