Real Time User Analytics API: Code Breakup of src/models/User.js
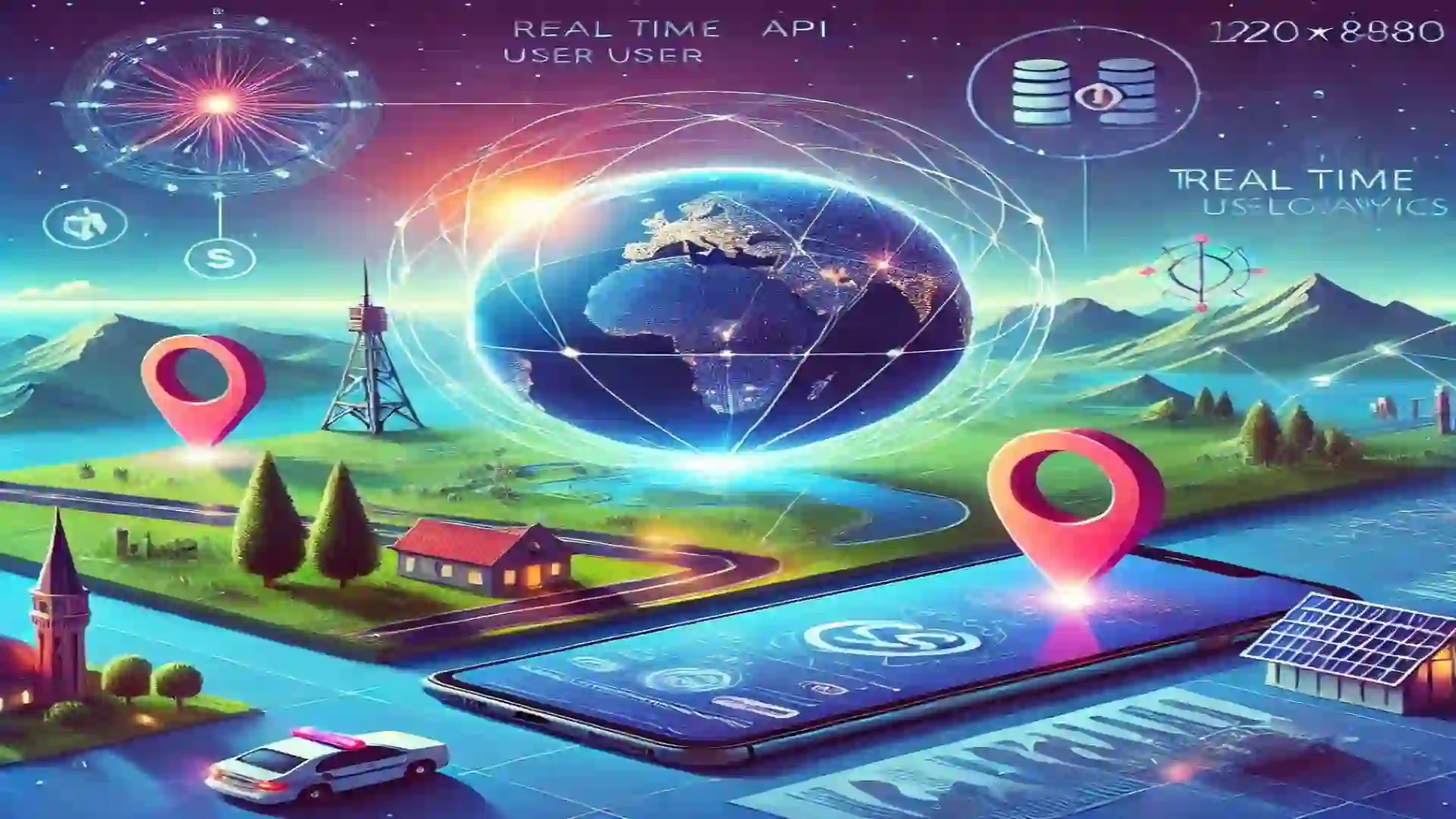
In modern web applications, tracking user information is essential for analytics, personalization, and security purposes.
One efficient way to handle such data is by using MongoDB with Mongoose, an elegant MongoDB object modeling tool for Node.js.
Let’s explore how the given code helps in creating a Mongoose schema to track user data.
Code Breakdown
1. Importing Mongoose
import mongoose from "mongoose";
- This line imports the Mongoose library, which provides a schema-based solution to model data for MongoDB.
- Mongoose acts as an abstraction layer, allowing developers to define schemas and interact with the database using an Object Data Modeling (ODM) approach.
2. Defining the Schema
const userSchema = new mongoose.Schema({
ip: { type: String, required: true },
browser: { type: String },
os: { type: String },
location: { type: Object },
timestamp: { type: Date, default: Date.now },
});
A schema in Mongoose defines the structure of documents in a MongoDB collection. Each field in the schema specifies:
- The data type it expects.
- Additional rules like whether it’s required or has default values.
Here’s what each field does:
ip
(String, Required):- Stores the IP address of the user.
required: true
ensures that this field must always be provided when a document is created.
browser
(String):- Stores the name or type of the browser the user is using (e.g., Chrome, Firefox).
os
(String):- Stores the operating system information (e.g., Windows, macOS, Linux).
location
(Object):- Stores location-related data about the user. This can include country, city, latitude, and longitude.
- No strict structure is imposed here, so this field can store any key-value pairs related to location.
timestamp
(Date, Default:Date.now
):- Records the time when the document is created.
default: Date.now
automatically assigns the current date and time if no value is provided.
3. Creating the Model
const User = mongoose.model("User", userSchema);
mongoose.model()
creates a model from the schema.- A model is a wrapper for the schema and provides an interface to interact with the corresponding MongoDB collection.
- The first argument,
"User"
, specifies the name of the collection. By default, Mongoose will pluralize it, so the collection name will beusers
. - The second argument is the schema object.
This model allows you to perform operations like:
- Creating documents (e.g., new user entries).
- Querying documents (e.g., finding users by IP).
- Updating documents (e.g., changing location data).
- Deleting documents (e.g., removing user entries).
4. Exporting the Model
export default User;
- This line exports the User model so it can be imported and used in other parts of the application.
- The
default
keyword ensures that the model is the default export of the module.
Example Use Case
Here’s how you might use this model in an application:
1. Importing the Model
import User from './path/to/your/model';
2. Creating a New User Document
const newUser = new User({
ip: "192.168.0.1",
browser: "Chrome",
os: "Windows",
location: { country: "India", city: "Mumbai" },
});
// Save to the database
newUser.save()
.then(() => console.log("User saved successfully"))
.catch((error) => console.error("Error saving user:", error));
3. Querying the Database
// Find all users who use Chrome
User.find({ browser: "Chrome" })
.then(users => console.log(users))
.catch(error => console.error(error));
4. Updating a Document
User.updateOne({ ip: "192.168.0.1" }, { os: "Linux" })
.then(() => console.log("User updated"))
.catch(error => console.error(error));
5. Deleting a Document
User.deleteOne({ ip: "192.168.0.1" })
.then(() => console.log("User deleted"))
.catch(error => console.error(error));
Key Features and Benefits of This Code
- Schema Enforcement:
- By defining a schema, you ensure consistency in your MongoDB collection. Only fields defined in the schema can be stored, preventing accidental mistakes.
- Default Values:
- Automatically setting a timestamp simplifies logging and tracking.
- Extensibility:
- New fields can easily be added to the schema if additional data needs to be stored in the future.
- Integration with MongoDB:
- Mongoose provides built-in methods for validation, middleware, and querying, making database operations easier and less error prone.
This code snippet provides a robust starting point for managing user-related data in a MongoDB collection.
Its use of Mongoose allows for flexibility, data integrity, and ease of interaction with the database.
By extending it with additional features like validations and middleware, it can be adapted to suit a wide range of application needs.